Creating your first jQuery plugin means that you’re no longer a beginner. You can now create something that you can use over and over again, or even package and sell to other developers.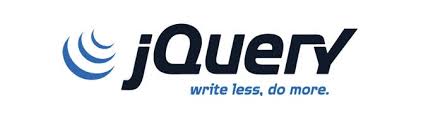
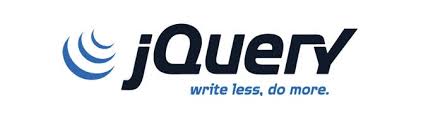
It may seem hard, but in reality creating a jQuery plugin is actually a simple process. In this article I’ll show you how simple by creating a simple tooltip plugin.
THE MARKUP
Any jQuery plugin requires some kind of markup and in our case all the plugin will need to be able to run properly is an anchor tag that has a tooltip data attribute.
We will make our plugin get the text we place inside the data-tooltip attribute and show that as the text in our tooltip. So the markup I will use in this example is minimal and it’s just a paragraph tag with a couple of anchor tags for testing like so:
<section>
<p>Your bones <a href="#" class="tooltip" data-tooltip="But mine do">don't break</a>. That's clear. Your cells react to bacteria and viruses differently than mine. You don't get sick, I do. That's also clear. But for some reason, you and I react the exact same way to water. We swallow it too fast, we choke. We get some in our lungs, we drown. However unreal it may seem, we are connected, you and I. We're on the <a href="#" class="tooltip" data-tooltip="just on opposite ends">same curve</a>.</p>
</section>
Eventually we’ll be able to call the plugin like so:
$('a.tooltip').tooltipMe();
After our markup is ready we only need to reference jQuery on our page and then create a .js file for our plugin and reference that as well. (Note that you should always reference jQuery first, or it won’t work.)
CREATING THE PLUGIN
The first thing we need to do is protect the $. Since this symbol is popular among some JavaScript libraries we need to make sure there aren’t any clashes with other libraries. So to do this we need to wrap all our code inside an immediately invoked function expression and pass jQuery as the parameter $:
(function ( $ ) {
//Our Code
}( jQuery ));
After this we need to name our plugin by creating its function and in this case I called the plugin TooltipMe but you can call it anything you want as long as you replace the name in the function and when you call the plugin inside the HTML file:
$.fn.tooltipMe = function() {
We should always remember to use the each method because the user may use this plugin on many elements on a single page.
After we did this we need to do some error checking to make sure what our user is trying to use is an anchor tag and if this passes we will start deciding what to do when the mouse hovers over our anchor tag:
this.each(function() {
if($(this).is('a')) {
After this, and assuming we can use the plugin because it has been called in an anchor tag, we need to start the hover function by saving the text inside the data-tooltip attribute and then creating our tooltip with all the necessary styles:
$(this).hover(function(e) {
var text = $(this).attr('data-tooltip');
$('<div id="tooltip"/>').appendTo($(this)).text(text).hide()
.css({
backgroundColor: '#000',
color: '#fff',
borderRadius: '3px',
opacity: 0.8,
position: 'absolute',
float: 'left'
}).fadeIn('slow');
},
You can see that after grabbing the text from the markup, I created the tooltip div itself appending it to anchor tag in which the tooltip will be placed then I hid it until the styles are added.
Using the .css method in jQuery I added all the necessary styles to our tooltip, in this case that meant the background-color , text color, border-radius,opacity and also position and float. Now that our styles are set and have the values we want we can fade the tooltip in using the fadeIn method , we can set the value of this to anything but I chose slow to give it more of a long effect so that the user can see the animation happening.
This code creates our tooltip but when we move away from the anchor tag it doesn’t disappear and to do that we need the second part of the hover function:
function() {
$('#tooltip').remove();
});
}
In this part all I did was remove the tooltip div when the user moves the mouse away from the anchor tag and the final curly brackets close the error checking to ensure the code is called on an anchor tag.
The final thing we need to do is add some top and left values to our div every time our mouse moves, and since our tooltip is has a position of absolute that will be easy using the mousemove function, like so:
$(this).mousemove(function(e) {
$('#tooltip').css({
top: e.pageY + 10,
left: e.pageX + 10
});
});
});
return this;
}
})(jQuery);
As you can see we set some values using e.pageY and e.pageX, these values give us the exact coordinates of our mouse and I added ten to both the values to not have the tooltip sit right on top of the mouse.
Having this done all left to do is close all the open functions and also add a return this at the near end to allow the user to chain our tooltip plugin , this is a good practice when it comes to creating jQuery plugins.
You can now call our plugin using:
$('a.tooltip').tooltipMe();
CONCLUSION
As you can see taking these small steps and creating a rather simple jQuery plugin is simpler than you may have thought and it doesn’t differ that much from the code we use on our everyday coding, we just need to take more in to account the error handling.
In the next article we’ll cover how to add options to our jQuery plugin allowing our users to change the defaults and replace them with the styles they wish to make it a more flexible plugin.
Post a Comment