In the first part of this tutorial we created a very simple jQuery plugin, but the main problem with what we created was that the user didn’t have any control over how the tooltip would appear, and in this second part we will create the necessary code to allow the user to change the defaults and replace them with their styles.
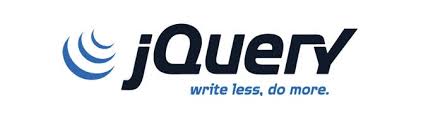
CREATING OUR OPTIONS
The first thing we need to do to allow the user to pass their own styles when calling the plugin is change the code we use to name the plugin, our code was:
$.fn.tooltipMe = function() {
In this line of code we need to pass options as the parameter of our function like so:
$.fn.tooltipMe = function(options) {
CREATING THE DEFAULTS
Since we added the parameter options we now need to create the defaults for our plugin and these defaults will run if the user runs the plugin without any parameters, our variable will be:
var defaults = $.extend({},{
background: '#000',
color: '#fff',
rounded: '3px',
opacity: 0.8
}, options);
This variable should be created right after we name our function and in this variable I first used the jQuery.extend to merge our defaults and the options the user types into an empty object and this way whatever the user types when calling the plugin will overwrite our defaults.
You can also create the variable with the defaults and then place it inside the extend like so:
var settings = {
background: '#000',
color: '#fff',
rounded: '3px',
opacity: 0.8
}
defaults = $.extend({},settings, options);
CHANGING THE .CSS
When this is finished our defaults are technically set, but we can’t used them just yet because if you look at our code all our CSS rules for the tooltip are hard coded, this means that no matter what we type when we call the plugin the CSS values won’t change and to make sure they do we need to make them dynamic:
.css({
backgroundColor: defaults.background,
color: defaults.color,
borderRadius: defaults.rounded,
opacity: defaults.opacity,
position: 'absolute',
float: 'left'
})
What we do in this code to make our defaults dynamic is get the default variable we defined at the top and set the CSS values equal to the defaults in that variable.
The final two options were still hard-coded because the functionality of the plugin depends on them.
With these options in place the full code for our plugin is:
(function($){
$.fn.tooltipMe = function(options) {
var defaults = $.extend({},{
background: '#000',
color: '#fff',
rounded: '3px',
opacity: 0.8
}, options);
this.each(function() {
if($(this).is('a')) {
$(this).hover(function(e) {
var text = $(this).attr('data-tooltip');
$('
').appendTo($(this)).text(text).hide()
.css({
backgroundColor: defaults.background,
color: defaults.color,
borderRadius: defaults.rounded,
opacity: defaults.opacity,
position: 'absolute',
float: 'left'
}).fadeIn('slow');
}, function() {
$('#tooltip').remove();
});
}
$(this).mousemove(function(e) {
$('#tooltip').css({
top: e.pageY + 10,
left: e.pageX+ 10
});
});
});
return this;
}
})(jQuery);
CALLING OUR PLUGIN
In the previous part of this tutorial we could only call the plugin like so:
$('a.tooltip').tooltipMe();
But now that our defaults are set, and we can override them, we can add parameters to this code to change the look of the plugin, like so:
$('a.tooltip').tooltipMe({
background: '#fff',
color: '#000',
rounded: '20px',
opacity: 1
});
In this example I made the background white, changed the text color to black, added a big border-radius and also made the opacity 1 so that it’s not semi-transparent. Since the defaults on our plugin were the black background, white text and small border-radius and transparency we completely changed its look and feel without touching its source code.
CONCLUSION
I hope that in this two part tutorial you saw and understood the basics of creating jQuery plugins.
This was just a demonstration of the power of creating jQuery plugins and hopefully was the starting boost you needed to write some jQuery plugins either for you or to spread online, and even if you get stuck one of the great things about jQuery is that it has a fabulous community so there is probably somebody out there who will help you fix the problem. One thing that we can never have too much of, is great jQuery plugins.
Post a Comment